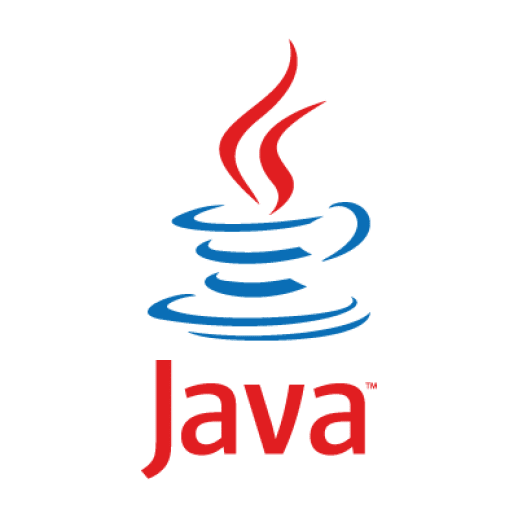
Hola! en este post voy a mostrar código de cómo crear un conversor de unidades numéricas de decimal a otros formatos. Leer el código, copiarlo, probarlo, estudiarlo, y aprender con él.
Hexadecimal
En este caso los números van a ir del 0 al 9 y a partir de ahí de la A a la F. por ejemplo B sería el 11.
Por aquí dejo el código:
public class Hexadecimal {
String convertir(int numero) {
String intermedio = "";
int numInt = 0;
while (numero > 15) {
numInt = numero
intermedio = intermedio + traducir(numInt);
numero = numero / 16;
}
intermedio = intermedio + traducir(numero);
intermedio = revertir(intermedio);
return intermedio;
}
String traducir(int numero) {
String str = "";
switch (numero) {
case 0:
str = "0";
break;
case 1:
str = "1";
break;
case 2:
str = "2";
break;
case 3:
str = "3";
break;
case 4:
str = "4";
break;
case 5:
str = "5";
break;
case 6:
str = "6";
break;
case 7:
str = "7";
break;
case 8:
str = "8";
break;
case 9:
str = "9";
break;
case 10:
str = "A";
break;
case 11:
str = "B";
break;
case 12:
str = "C";
break;
case 13:
str = "D";
break;
case 14:
str = "E";
break;
case 15:
str = "F";
break;
}
return str;
}
String revertir(String str) {
String intermedio = "";
for (int i = str.length() - 1; i >= 0; i--) {
intermedio = intermedio + str.charAt(i);
}
return intermedio;
}
}
Binario
En este sistema numérico sólo existen 2 números el cero y el uno, y el código queda así:
public class Binario {
String convertir(int numero) {
String intermedio = "";
int numInt = 0;
while (numero > 1) {
numInt = numero
intermedio = intermedio + numInt;
numero = numero / 2;
}
intermedio = intermedio + numero;
intermedio = revertir(intermedio);
return intermedio;
}
String revertir(String str) {
String intermedio = "";
for (int i = str.length() - 1; i >= 0; i--) {
intermedio = intermedio + str.charAt(i);
}
return intermedio;
}
}
Octal
En este sistema numérico tiene un sistema del 0 al 7, después se pone un acarreo con otro 0. Por aquí dejo el código:
public class Octal {
String convertir(int numero) {
String intermedio = "";
int numInt = 0;
while (numero > 7) {
numInt = numero
intermedio = intermedio + numInt;
numero = numero / 8;
}
intermedio = intermedio + numero;
intermedio = revertir(intermedio);
return intermedio;
}
String revertir(String str) {
String intermedio = "";
for (int i = str.length() - 1; i >= 0; i--) {
intermedio = intermedio + str.charAt(i);
}
return intermedio;
}
}
Vigesimal
Aquí hay 20 números, la verdad es un sistema numérico muy interesante, hacia adelante del 9 se empieza a contar con el abecedario hasta la J, por aquí dejo el código:
public class Vigesimal {
String convertir(int numero) {
String intermedio = "";
int numInt = 0;
while (numero > 19) {
numInt = numero
intermedio = intermedio + traducir(numInt);
numero = numero / 20;
}
intermedio = intermedio +traducir( numero);
intermedio = revertir(intermedio);
return intermedio;
}
String traducir(int numero) {
String str = "";
switch (numero) {
case 0:
str = "0";
break;
case 1:
str = "1";
break;
case 2:
str = "2";
break;
case 3:
str = "3";
break;
case 4:
str = "4";
break;
case 5:
str = "5";
break;
case 6:
str = "6";
break;
case 7:
str = "7";
break;
case 8:
str = "8";
break;
case 9:
str = "9";
break;
case 10:
str = "A";
break;
case 11:
str = "B";
break;
case 12:
str = "C";
break;
case 13:
str = "D";
break;
case 14:
str = "E";
break;
case 15:
str = "F";
break;
case 16:
str = "G";
break;
case 17:
str = "H";
break;
case 18:
str = "I";
break;
case 19:
str = "J";
break;
}
return str;
}
String revertir(String str) {
String intermedio = "";
for (int i = str.length() - 1; i >= 0; i--) {
intermedio = intermedio + str.charAt(i);
}
return intermedio;
}
}
El Programa principal
En el Main deberemos crear un menú, en el cual se pida un número y se llame a las funciones necesarias de Java para hacer las conversiones. Por aquí dejo el código:
public class Main {
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
// TODO code application logic here
Scanner sc = new Scanner(System.in);
int numero = 0;
String convertido;
int eleccion = 0;
//declaramos las clases
Hexadecimal hx = new Hexadecimal();
Binario bn = new Binario();
Vigesimal vg = new Vigesimal();
Octal oc = new Octal();
while (eleccion != 4) {
System.out.println("Dígame número a convertir");
numero = sc.nextInt();
System.out.println("El valor de " + numero + " en hexadecimal es " + hx.convertir(numero));
System.out.println("El valor de " + numero + " en binario es " + bn.convertir(numero));
System.out.println("El valor de " + numero + " en vigesimal es " + vg.convertir(numero));
System.out.println("El valor de " + numero + " en octal es " + oc.convertir(numero));
System.out.println("Elige cualquier numero para continuar");
System.out.println("Elige 4 para salir");
eleccion = sc.nextInt();
}
}
}
Conclusión:
Espero que os haya gustado mi código, si necesitas ayuda con Java puedes dejarmelo en la selección de contacto, estés en instituto, universidad, o simplemente quieres aprender de forma autodidacta. Sin más me despido, un saludo y hasta la próxima.